Top 10 Most Useful Flutter Widgets
20 October 2024
Flutter has rapidly become one of the most popular frameworks for building cross-platform applications. One of the key reasons behind this popularity is its extensive ecosystem of packages that simplify the development process. In this article, we'll explore Best Flutter Widgets and learn integrating widgets with some best practices to follow.
What are Flutter Widgets?
Before we continue with the list, let's clarify what widgets are. In Flutter, everything is a widget, from the simplest text element to complex layouts it's a library for all your UI needs. They are the building blocks that compose your app's user interface.
Many of you are familiar with widgets like Container, image, row-column text, etc, as they are most commonly used across the flutter community! Ever wonder how many types of widgets are there in Flutter! We will cover some of the lesser-known yet efficient flutter widgets list that can improve your app's user experience.
Yes, these widgets are easy to use and install but if you want to use them efficiently then you should consider to Hire Flutter Developer.
1. AlertDialog
First on the list is AlertDialog. When you need to grab your users' attention, alert them to something important, or guide them through a specific action, then AlertDialogs are your go-to tool. These pop-up windows will provide a clear way to communicate with your users.
Once implemented, the most basic dialog box will have some content along with buttons and the most advanced ones can have animated text, images, or anything design-specific.
To make sure it is clear to the users what they are getting the alert about, make the action buttons simple and easy to understand, so they can proceed further smoothly.
Material AlertDialogs are designed for Android-style apps. They are built like a card so you can change their shape, size, and even color.
Where on the other hand CupertinoAlertDialogs are designed for iOS-style apps. They have a slightly different look and feel, but they work similarly to Material AlertDialogs. To show this dialog box simply pair it with showCupertinoDialog and showDialog and that’s it now you can make Dialogs similar to Material World
Now all you need to do is render it for iOS.
2. DropdownMenu
The DropdownMenu is a useful widget for creating user interfaces, especially when you have a lot of options to choose from. Unlike smaller lists that can be shown with radio buttons, the DropdownMenu fits neatly into a text field and lets users pick from a larger selection. When a user selects an item, the text field fills in with their choice.
To use a DropdownMenu, we need to create it and provide a list of items called DropdownMenuEntry, which includes a name (label) that users see and a value that stores the actual data. This widget also has a search feature, making it easy to find specific options within a long list, and you can turn this search feature on or off. Additionally, the DropdownMenu allows for styling and accessibility features, which helps improve the user experience.
To un your own sample code:
flutter create --sample=material.DropdownMenu.1 mysample
3. Circular progress indicator and linear program indicator
The CircularProgressIndicator and LinearProgressIndicator widgets in Flutter are important tools to showcase the progress of your MaterialApp.
Both CircularProgressIndicator and LinearProgressIndicator widgets can be used to show your progress in a circular or linear manner. These APIs are very similar to each other and both can indicate the amount of progress that the app is making on a task.
You can also change the color value of the progress indicator as well, this value can be animated as well but if you want a single color then use AlwaysStoppedAnimation, and if you want to fancy then you can also create animation by using color animations.
If you want a bar or a circle as your progress indicator, set the value to showcase the process of completion.
According to your design, you may need to call setState or wrap your indicator in an AnimatedBulider, streamBuilder, or any other builder with the updated value. If you want a CircularProgressIndicator then you need to use the strokeWidth, to make your indicator as thick or thin as you want.
That's it you are all set to check whether your app is making ideal progress or not.
4. Flutter- slidable
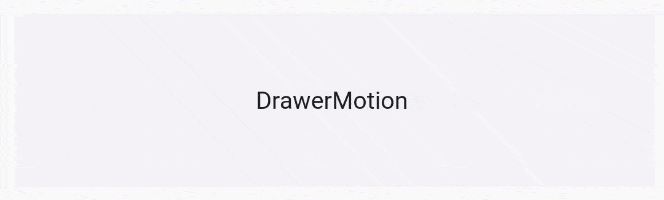
The flutter_slidable package provides a convenient way to implement the common swipe-to-reveal-actions pattern in mobile UI design.
By wrapping a ListTile (or any other widget) in a Slidable widget, you can define custom actions that are revealed when the user swipes the item in a specific direction.
The actionPane property determines how the actions are displayed, with options like SlidableScrollActionPane for scrolling actions from off-screen.
The actions parameter takes a list of widgets representing the available actions, and the IconSlideAction helper simplifies the creation of these actions.
Finally, the actionExtentRatio property specifies the fraction of space each action should occupy, ensuring they are all visible.
5. Grid view
The GridView widget in Flutter provides an efficient way to create grid layouts with multiple rows and columns. It offers various constructors, with the count constructor being one of the most commonly used.
To create a GridView using the count constructor, you first need to specify the list of items you want to display in the grid. Then, you can control the number of items displayed across each row by setting the crossAxisCount property. Setting it to 1 will result in a list-like layout while setting it to a higher value (e.g., 10) will create a more traditional grid arrangement.
For additional customization, you can use the mainAxisSpacing and crossAxisSpacing properties to adjust the spacing between rows and columns, respectively. This allows you to create grids with varying levels of density and visual appeal.
Similar to ListView, GridView supports scrolling, enabling users to navigate through large datasets easily.
6. OverlayPortal
When building apps with Flutter, you often place child widgets directly inside their parent widgets to create a unified look. However, there are times when you want a widget that is related to its parent but appears separate, like a tooltip or a floating menu. In such cases, you can use the OverlayPortal widget.
The OverlayPortal allows you to display a widget anywhere on the screen, overlaying other existing widgets. To use it, you simply add the OverlayPortal as a child to the widget you want it to be associated with, such as a button. You also need to provide an OverlayPortal controller to control when the overlay appears or disappears. The overlay content is created using a callback function that returns the widget you want to show.
When you press the associated button, the overlay will display. You can also add a child widget to the OverlayPortal, which will show up as part of its parent. If you want to control the position of the overlay, you can use a positioned widget to specify where it should appear.
One key advantage of OverlayPortal over OverlayEntry is that it inherits its build context from its parent, meaning it will also inherit styles and themes. So, the next time you need a widget that is logically connected to its parent but visually distinct, consider using OverlayPortal.
7. Mouse region
MouseRegion is a Flutter widget that allows you to detect mouse interactions within a specific area of your application. This is particularly useful for desktop or web applications where users can interact with the screen using a mouse.
If you want to use this particular widget just make sure to use a widget that is aware of mice and wrap it in MouseRegion, now you will know if the mouse is in the region.
Make sure to give some indication of a mouse to the users that can be done by changing the pointer to something else with the cursor property
Most of the cursors are defined in the SystemMouseCursors class. Then comes the callbacks you can set up according to your preferences.
That's it you are all set you can use this widget either for gaming apps, maps, or even for your custom interactions.
8. Physical model
The PhysicalModel widget is a powerful tool for creating realistic and visually appealing shadows in Flutter applications. By wrapping any widget in a PhysicalModel, you can add a layer underneath it that represents the object casting a shadow, giving your UI elements more of a 3D feel.
To use PhysicalModel, simply wrap your desired widget in the PhysicalModel constructor and provide a color for the shadow. By default, the elevation of the PhysicalModel is zero, so you'll need to increase it to create a visible shadow. You can also customize the shape, roundness, and color of the shadow to achieve the desired effect.
It's important to note that PhysicalModel doesn't cause the child widget itself to cast a shadow. Instead, it creates a separate layer beneath the child that represents the shadow. This allows you to manipulate the shadow's properties independently of the child widget.
PhysicalModel is particularly useful for creating custom shadow effects and is used internally by other widgets like Material to generate material-style shadows.
9. Scroll bar
This widget is mostly used when you want to show the user how far they’ve scrolled.
To get started and to show a scrollbar use the widget you guessed it “scrollbar”.
Now you can use the scrollbar horizontally or vertically and also style them as your design requirements.
This is the most important step to wrap the scrollbar around any scrollable widget making sure that the scrollable widget is finite. So the widget knows how far to go down.
That's it you are all set!
Now it's up to you if you decide to show the track or not, but if you want to show the track use showOnHover.
10. CallbackShortcuts
If you want to quickly add keyboard shortcuts to your Flutter app, you can use CallbackShortcuts. This widget allows you to map specific keyboard combinations directly to actions in your app without the need for the more complex shortcuts, actions, and intents system.
Using CallbackShortcuts is straightforward. Most interactive widgets, like buttons and switches, already have built-in support for handling key events. If you're working with a widget that doesn't, such as a text widget, you just need to add a focus widget to enable it to receive key events.
Once your widget can gain focus, you can wrap it in a CallbackShortcuts widget to define your keyboard shortcuts. In the bindings parameter, you provide a map linking ShortcutActivators—which helps you specify the key combinations—to the corresponding callback functions that should be triggered when those shortcuts are pressed.
For example, if you want to capture the plus key, you can use a CharacterActivator and specify that you want to watch for the "+" character. If you need to capture a key combination with a modifier, like Control + R, you would create a SingleActivator, specify the R key, and set the control parameter to true.
In summary, define your activation keys and their corresponding callbacks in a map, and you're all set to implement keyboard shortcuts in your app! For more details about CallbackShortcuts and other Flutter widgets, check out flutter.dev.
Wrapping Up
Flutter has many great tools for building apps, and we focus on ones you might not know about, like AlertDialog, DropdownMenu, CircularProgressIndicator, and CallbackShortcuts. Each widget is explained in simple terms, showing what it does and how to use it. Whether you're creating a complex app or a simple one, these widgets can help improve the user experience. If you are confused between technology and don't know which one would be the best for you then we offer free consultancy to help you get a better idea on why choose flutter if you're interested in adding these features to your app.
WRITTEN BY

Pratik Butani
Pratik is a mobile app developer who makes both iOS and Android apps look and feel amazing using Flutter. He brings ideas to life with clean, smooth, and functional apps that users love.
WRITTEN BY
Pratik Butani
Pratik is a mobile app developer who makes both iOS and Android apps look and feel amazing using Flutter. He brings ideas to life with clean, smooth, and functional apps that users love.
More
An interesting read? Here is more related to it.
Making IT Possible
Making IT Possible
Making IT Possible
Making IT Possible
Making IT Possible
Making IT Possible
India (HQ)
201, iSquare Corporate Park, Science City Road, Ahmedabad-380060, Gujarat, India
For Sales
[email protected]
Looking For Jobs
Apply Now