Next.js vs. React: Which Front-End Framework to Choose? š
22 November 2024
Table of contents
Planning to build a powerful front-end for your website but unsure about Next.js vs React? Or are you a budding developer looking to master front-end development but confused about which technology to choose? Both technologies have their unique strengths, and picking the right one can make a big difference.

Market Share and Adoption
According to Stack Overflow's 2023 Developer Survey, React remains the most popular front-end framework, with many developers using it. Next.js, while gaining traction, still has a smaller market share compared to React. However, its adoption rate is growing rapidly due to its performance benefits and developer experience.
Popularity Metrics
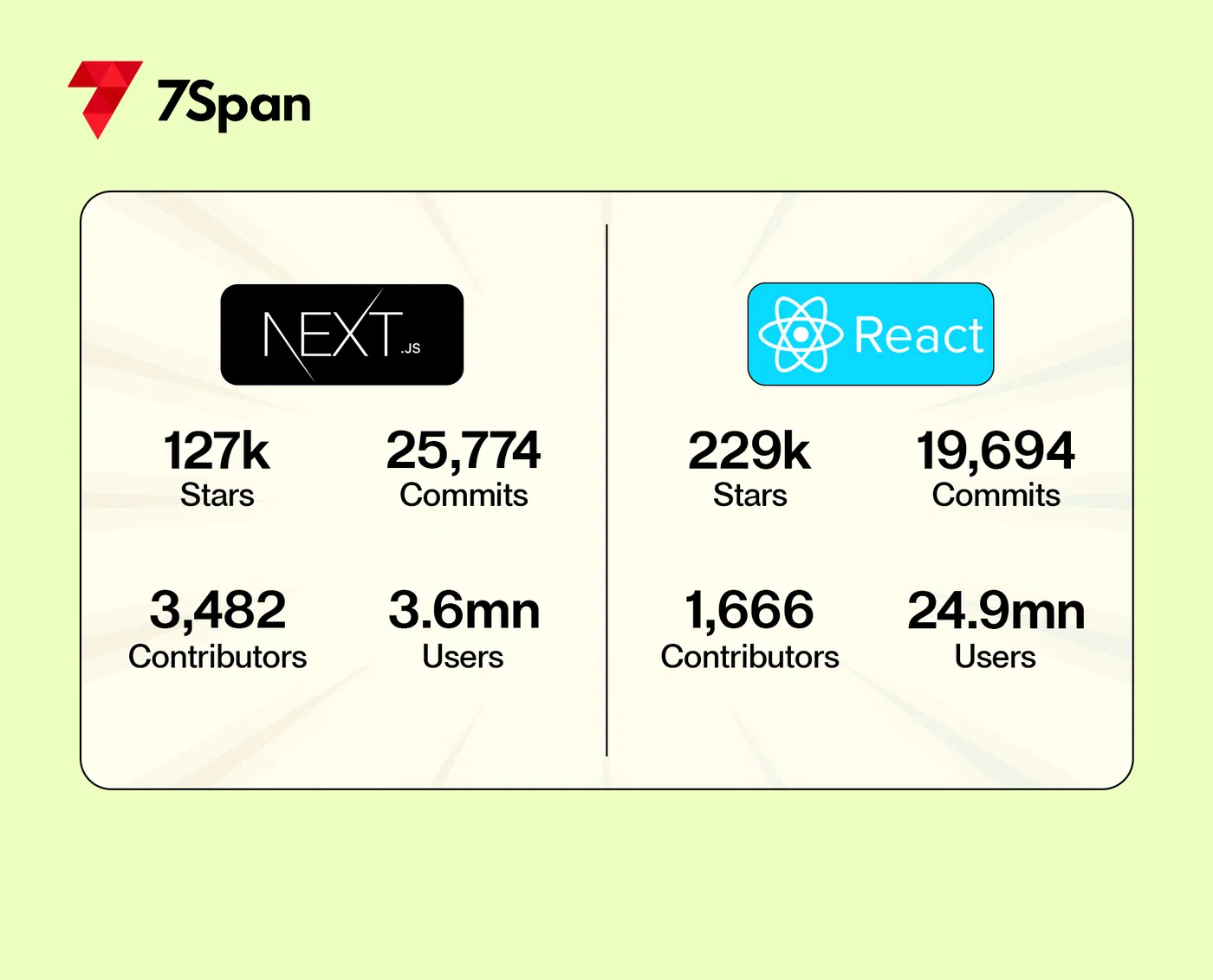
Real-world Examples
To illustrate the differences, here are some popular websites built with React and Next.js:

Letās look at these technologies in a bit more detail.
What is React? āļø

Built by Facebook, React is a JavaScript library for building user interfaces. It's like LEGO bricks for your website or app, letting you create complex structures from simple components.
React is all about creating reusable components that can be combined to form complex applications.
Key Features of React
Component-Based Architecture:
One of the fundamental strengths of React is its component-based architecture. This approach enables developers to break down the user interface into reusable components, each encapsulating its own logic, styling, and markup. By structuring your application in this way, you improve code organization and maintainability.
Instead of dealing with a monolithic codebase, developers can focus on smaller, independent components that can be easily updated, tested, and reused across different parts of the application.
This modularity not only improves collaboration among team members but also facilitates a more efficient development process, especially for larger projects.
JSX:
React utilizes JSX, a syntax extension for JavaScript that closely resembles HTML. This unique feature allows developers to write components using a familiar and intuitive syntax, which can significantly simplify the process of building user interfaces.
By enabling developers to create UI elements with both logic and markup in a single file, JSX streamlines the development experience and reduces context switching.
Additionally, JSX is compiled into JavaScript at build time, ensuring compatibility with modern browsers while allowing developers to benefit from a more readable and expressive syntax. This can be particularly advantageous for teams looking to onboard new developers quickly or those transitioning from traditional HTML-based frameworks.
Virtual DOM:
Another key aspect of React is its use of the Virtual DOM, which is a lightweight representation of the actual Document Object Model (DOM). When changes are made to the UI, they are first applied to the Virtual DOM, where React can efficiently calculate the minimum number of changes needed to update the actual DOM.
This process minimizes direct interactions with the DOM, which can be slow and resource-intensive, thereby enhancing performance significantly. For developers concerned about speed and responsiveness in their applications, especially those that require real-time updates or have dynamically changing data, Reactās Virtual DOM offers a compelling advantage that can lead to a smoother user experience.
Built-in State Management:
React also provides built-in state management mechanisms that are essential for tracking the application's changing data over time. This feature allows developers to manage component states effectively, ensuring that UI updates in response to user interactions or data changes are seamless and efficient.
By incorporating state management directly into the framework, React alleviates the need for external libraries for simple state use cases, making it easier and more intuitive for developers to track and manage application states.
This can be particularly beneficial for projects that require quick prototyping or for teams looking to minimize the complexity of their tech stack.
When to Use React š¤
- Small to medium-sized projects: React offers flexibility and control for smaller projects.
- Complex user interfaces: React's component-based architecture excels in building intricate UIs.
- Single-page applications (SPAs): React is well-suited for SPAs with dynamic interactions.
- Maximum flexibility: If you need full control over the development process, React is the way to go.
How to get started with React
(This is for new developers who are trying to figure out which framework to learn and master.)
To get started with React, you can create a simple React application using Create React App, which sets up everything you need to begin developing. Below is a step-by-step guide along with code snippets.
Step 1: Install Node.js
Make sure you have Node.js installed on your machine. You can download it from [nodejs.org](https://nodejs.org/)
Step 2: Create a New React Application
Open your terminal and run the following command to create a new React app:
```bash
npx create-react-app my-app
```
Replace `my-app` with your desired project name.
Step 3: Navigate to Your Project Directory
Change into your project directory:
```bash
cd my-app
```
Step 4: Start the Development Server
Run the following command to start the development server:
```bash
npm start
```
This will open your new React app in your default web browser at `http://localhost:3000`.
Step 5: Modify the App Component
Open the `src/App.js` file in your favorite code editor and replace its content with the following code to create a simple greeting component:
```javascript
const App = () => {
Ā Ā Ā Ā return (
Ā Ā Ā Ā Ā Ā Ā Ā <div className="flex flex-col items-center justify-center min-h-screen bg-gray-100">
Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā <h1 className="text-4xl">Welcome to My React App!</h1>
Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā <p className="mt-4">This is a simple React application.</p>
Ā Ā Ā Ā Ā Ā Ā Ā </div>
Ā Ā Ā Ā );
};
export default App;
```
import React from "react";
Step 6: Install Tailwind CSS (Optional)
If you want to use Tailwind CSS for styling, you can install it by following these steps:
1. Install Tailwind via npm:
Ā Ā Ā ```bash
Ā Ā Ā npm install -D tailwindcss postcss autoprefixer
Ā Ā Ā ```
2. Create the configuration files:
Ā Ā Ā ```bash
Ā Ā Ā npx tailwindcss init -p
Ā Ā Ā ```
3. Configure your `tailwind.config.js` file:
Ā Ā Ā ```javascript
Ā Ā Ā module.exports = {
Ā Ā Ā Ā Ā content: ["./src/**/*.{js,jsx,ts,tsx}", "./public/index.html"],
Ā Ā Ā Ā Ā theme: {
Ā Ā Ā Ā Ā Ā Ā extend: {},
Ā Ā Ā Ā Ā },
Ā Ā Ā Ā Ā plugins: [],
Ā Ā Ā };
Ā Ā Ā ```
4. Add the Tailwind directives to your `src/index.css` file:
Ā Ā Ā ```css
Ā Ā Ā @tailwind base;
Ā Ā Ā @tailwind components;
Ā Ā Ā @tailwind utilities;
Ā Ā Ā ```
Step 7: View Your Application
After making these changes, your application should automatically reload. You should see your greeting message styled with Tailwind CSS.
You now have a basic React application set up and running! From here, you can start building more complex components and exploring React's features.
P.S. Check out these beginner-friendly React projects to help you start building your portfolio!
What is Next.js? š

Next.js is built on top of React. It takes React's functionality and adds features like server-side rendering (SSR), static site generation (SSG), and optimized image handling. It's perfect for creating fast, SEO-friendly websites. It's like adding a turbocharger to your React application.
Key Features of Next.js
Server-Side Rendering (SSR):
One of the standout features of Next.js is its ability to perform Server-Side Rendering (SSR). This means that pages are rendered on the server rather than in the browser, which can significantly enhance your website's performance.
By generating pages on the server, SSR improves search engine optimization (SEO) since search engines can easily crawl and index the content. Additionally, it leads to faster initial load times for users, as they receive fully rendered HTML on their first visit.
This can also improve social sharing, as the shared links will display rich previews, making your content more engaging and clickable.
Static Site Generation (SSG):
Next.js also supports Static Site Generation (SSG), which allows you to pre-render pages at build time. This method provides lightning-fast performance because the pages are served as static files, eliminating the need for server processing on each request.
SSG is particularly cost-effective, as it reduces server load and can lead to lower hosting costs. For websites that donāt require frequent updates, SSG is an excellent choice, ensuring that users enjoy a fast and responsive experience while keeping your infrastructure efficient.
Image Optimization:
Another significant advantage of Next.js is its built-in image optimization feature. This automatically optimizes images for different screen sizes and formats, ensuring that your website delivers the best possible performance without sacrificing quality.
By serving appropriately sized images based on the userās device, Next.js helps reduce load times and improve overall user experience.
This is particularly important in todayās mobile-first world, where users expect fast-loading pages and seamless browsing experiences.
API Routes:
Next.js makes it easy to create backend API endpoints directly within your application using API routes. This feature allows you to build a full-stack application without needing a separate backend server.
By defining API routes, you can handle requests, fetch data, and interact with databases all within the same codebase.
This can simplify your development process, as you can manage both the front end and backend in one place, making it easier to maintain and deploy your application.
Incremental Static Regeneration (ISR):
Incremental Static Regeneration (ISR) is another powerful feature of Next.js that allows you to update specific parts of a static site without having to rebuild the entire application.
This means you can keep your content fresh and up-to-date while still benefiting from the performance advantages of static site generation. ISR is particularly useful for websites that require frequent updates, such as blogs or e-commerce sites, where certain pages need to reflect the latest information without sacrificing speed or efficiency.
Automatic Code Splitting:
Next.js also excels in optimizing your applicationās bundle size through automatic code splitting. This feature divides your code into smaller chunks, loading only the necessary parts for each page.
By ensuring that users only download what they need, Next.js improves loading times and enhances the overall performance of your application.
This is especially beneficial for larger applications, as it helps reduce the initial load time and provides a smoother experience for users as they navigate through different pages.
When to Use Next.js š¤
- SEO-focused websites: Next.js's SSR and SSG capabilities improve search engine visibility.
- High-performance applications: Next.js delivers faster load times and better user experiences.
- Websites with dynamic content: Next.js can handle data fetching and rendering efficiently.
- Accelerated development: Built-in features and conventions streamline the development process.
How to get started with Next.js
To get started with Next.js, you can follow these steps to set up a new Next.js project and create a simple page. Below is a basic example of a Next.js application.
1. Install Node.js:
Make sure you have Node.js installed on your machine. You can download it from [nodejs. org](https://nodejs.org/).
2. Create a New Next.js App:
Open your terminal and run the following command to create a new Next.js application:
```bash
npx create-next-app@latest my-next-app
```
Replace `my-next-app` with your desired project name.
3. Navigate to Your Project Directory:
```bash
cd my-next-app
```
4. Start the Development Server:
```bash
npm run dev
```
This will start the development server, and you can view your app at `http://localhost:3000`.
5. Create a Simple Page:
Open the `pages/index.js` file and modify it to create a simple homepage. Hereās an example code snippet:
```javascript
import Head from 'next/head';
export default function Home() {
return (
<div>
<Head>
<title>My Next.js App</title>
<meta name="description" content="Generated by create next app" />
</Head>
<main className="flex flex-col items-center justify-center min-h-screen">
<h1 className="text-4xl font-bold">Welcome to My Next.js App!</h1>
<p className="mt-4">This is a simple Next.js application.</p>
</main>
</div>
);
}
```
6. Install Tailwind CSS (Optional):
If you want to use Tailwind CSS for styling, you can install it by following these steps:
Ā Ā Ā - Install Tailwind CSS via npm:
Ā Ā Ā ```bash
Ā Ā Ā npm install -D tailwindcss postcss autoprefixer
Ā Ā Ā ```
Ā Ā Ā - Initialize Tailwind CSS:
Ā Ā Ā ```bash
Ā Ā Ā npx tailwindcss init -p
Ā Ā Ā ```
Ā Ā Ā - Configure your `tailwind.config.js` file:
Ā Ā Ā ```javascript
Ā Ā Ā /** @type {import('tailwindcss').Config} */
Ā Ā Ā module.exports = {
Ā Ā Ā Ā Ā content: [
Ā Ā Ā Ā Ā Ā Ā "./pages/**/*.{js,ts,jsx,tsx}",
Ā Ā Ā Ā Ā Ā Ā "./components/**/*.{js,ts,jsx,tsx}",
Ā Ā Ā Ā Ā ],
Ā Ā Ā Ā Ā theme: {
Ā Ā Ā Ā Ā Ā Ā extend: {},
Ā Ā Ā Ā Ā },
Ā Ā Ā Ā Ā plugins: [],
Ā Ā Ā }
Ā Ā Ā ```
Ā Ā Ā - Add the Tailwind directives to your `styles/globals.css` file:
Ā Ā Ā ```css
Ā Ā Ā @tailwind base;
Ā Ā Ā @tailwind components;
Ā Ā Ā @tailwind utilities;
Ā Ā Ā ```
7. Build and Deploy:
When you're ready to deploy your application, you can build it using:
Ā Ā Ā ```bash
Ā Ā Ā npm run build
Ā Ā Ā ```
Then, you can deploy it to platforms like Vercel or Netlify.
This should give you a solid start with Next.js! Here are some projects to get you started!
React vs Next.js: Use Cases
E-commerce Platforms
- React: Well-suited for building complex e-commerce storefronts with interactive features like product filters, shopping carts, and checkout processes. But lacks in website performance.
- Next.js: Ideal for e-commerce platforms that prioritize SEO and fast load times. SSR can improve product page indexing, while image optimization can enhance user experience. Next.js's ability to handle dynamic product data and generate static pages makes it a strong contender for e-commerce.
Enterprise Applications
- React: Excellent choice for building large-scale enterprise applications with complex user interfaces and data management requirements. Its flexibility and component-based approach facilitate the creation of scalable and maintainable solutions.
- Next.js: Can be used for enterprise applications that require SEO, fast initial load times, or server-side data fetching. However, for extremely complex enterprise applications with extensive interactions, React might offer more flexibility.
Content-Heavy Websites (Blogs, News, Portfolios)
- React: Suitable for creating dynamic and interactive content-heavy websites with features like infinite scrolling, real-time updates, and user-generated content.
- Next.js: Ideal for content-heavy websites that prioritize SEO and performance. SSG can be used to pre-render content, improving load times and search engine visibility.
Marketing Landing Pages
- React: Can be used for creating interactive and engaging landing pages with animations and dynamic content.
- Next.js: Offers advantages in terms of SEO and performance, making it a good choice for landing pages that need to rank well in search results.
Real-Time Applications (Chat, Collaboration Tools)
- React: Well-suited for building real-time applications due to its ability to efficiently update the UI in response to data changes.
- Next.js: While possible, might not be the optimal choice for real-time applications that require constant updates and low latency. React's flexibility and component-based architecture are better suited for this type of application.
Next.js vs React: Key Differences
Now that weāve explored what Next.js and React are and their used cases, letās discuss their key differences!
Rendering š¦
- React: Primarily relies on client-side rendering (CSR), meaning the browser downloads the JavaScript code and builds the UI on the user's device.
- Next.js: Offers both client-side rendering (CSR) and server-side rendering (SSR). SSR renders the page on the server and sends the HTML to the browser, improving initial load times and SEO.
Features āļø
- React: As a core library, React provides the building blocks for creating user interfaces. Additional features like routing, state management, and data fetching are often handled by external libraries.
- Next.js: Built on top of React, Next.js offers built-in features such as file-system-based routing, image optimization, data fetching and API routes, and streamlining development.
Performance š
- React: Performance is heavily dependent on the developer's implementation, with techniques like component optimization and state management crucial for achieving good performance.
- Next.js: Generally offers better performance out-of-the-box due to features like automatic code splitting, image optimization, and SSR, which can significantly improve initial load times and overall responsiveness.
Other Key Differences ā”ļø
- Routing: Next.js comes with built-in file-system-based routing, making it easy to manage your routes. React requires a separate routing library like React Router.
- Data Fetching: Next.js provides getStaticProps and getServerSideProps for fetching data, while React relies on libraries like fetch or axios.
- API Routes: Next.js allows you to create API endpoints directly within your application, simplifying backend development.
- Deployment: React apps can be deployed on any static site hosting, but Next.js is optimized for platforms like Vercel.
- File Structure: React doesn't have a specific file structure, while Next.js promotes a convention-over-configuration approach.
- Initial Setup: React often requires more setup for configuration, while Next.js comes with useful defaults out of the box.
Still confused? Don't worry! Both React and Next.js have huge communities š„ and tons of resources to help you learn.
Next.js vs React: Which One to Choose? š¤
Ultimately, the choice between Next.js and React depends on your project's specific requirements as discussed earlier.
Choose React if:
- You have a small to medium-sized project.
- You need maximum flexibility and control over the development process.
- You're building a complex single-page application.
Choose Next.js if:
- You prioritize performance and SEO.
- You need to build a website with dynamic content.
- You want to accelerate development with built-in features.
Additional Considerations
- Ecosystem: Both React and Next.js have extensive ecosystems with a wide range of libraries and tools available.
- Deployment: While React apps can be deployed on various platforms, Next.js integrates seamlessly with platforms like Vercel.
- Learning curve: React generally has a lower learning curve, while Next.js might require additional time to master its features.
Remember š§ : You can always start with React and gradually introduce Next.js features as your project grows. šāØ
Conclusion
Both React and Next.js are powerful tools for building modern web applications. By carefully considering your project's requirements and the strengths of each framework, you can make an informed decision and achieve your development goals.
Need more help? Hire Next.js Developers and React Developers to gain clarity on your projects! šš”
WRITTEN BY

Abhishek Vasvelia
Abhishek is a Next.js expert who creates fast, high-performance websites. With a keen eye for detail, he make sures every site loads quickly and runs smoothly.
WRITTEN BY
Abhishek Vasvelia
Abhishek is a Next.js expert who creates fast, high-performance websites. With a keen eye for detail, he make sures every site loads quickly and runs smoothly.
More
An interesting read? Here is more related to it.
Making IT Possible
Making IT Possible
Making IT Possible
Making IT Possible
Making IT Possible
Making IT Possible
India (HQ)
201, iSquare Corporate Park, Science City Road, Ahmedabad-380060, Gujarat, India
For Sales
[email protected]
Looking For Jobs
Apply Now