Top 10 Flutter Packages
27 November 2024
Table of contents
What are Flutter Packages?
Types of Flutter Packages
Packages:
Plugins:
Top 10 Flutter Packages
1. path_provider
2. flutter_animate
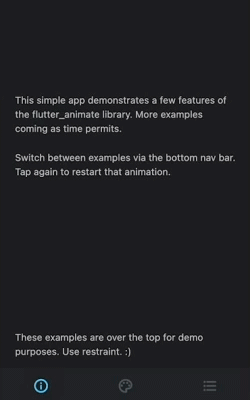
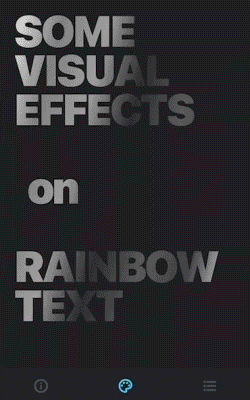
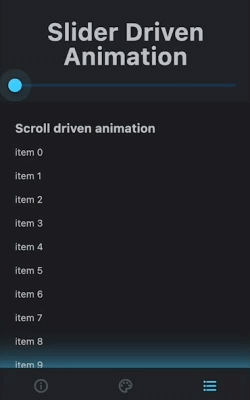
3. google_fonts
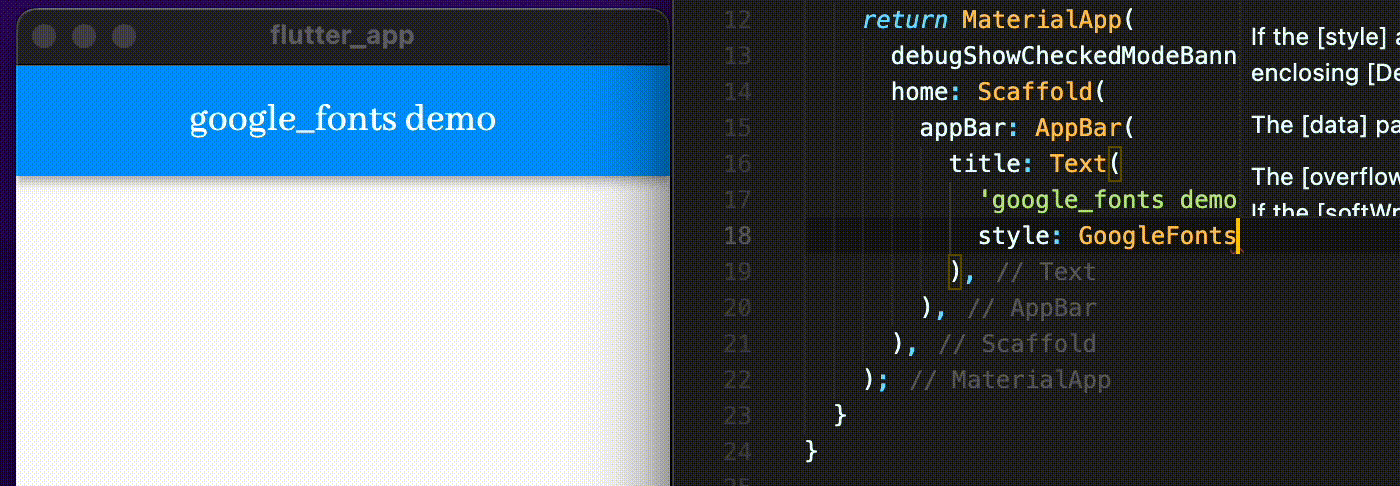
4. url_launcher
import 'package:flutter/material.dart';
import 'package:url_launcher/url_launcher.dart';
final Uri _url = Uri.parse('https://7Span.com');
void main() => runApp(
const MaterialApp(
home: Material(
child: Center(
child: ElevatedButton(
onPressed: _launchUrl,
child: Text('Show 7Span homepage'),
),
),
),
),
);
Future<void> _launchUrl() async {
if (!await launchUrl(_url)) {
throw Exception('Could not launch $_url');
}
}
5. shared_preferences
6. flutter_lints
7. location
8. cached_network_image
9. sensors_plus
10. flutter_slidable
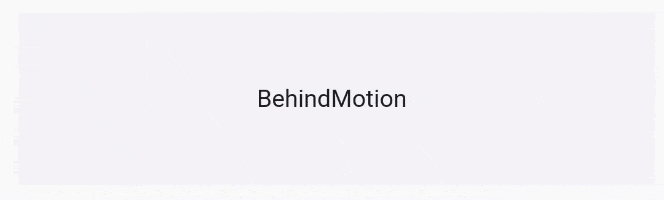
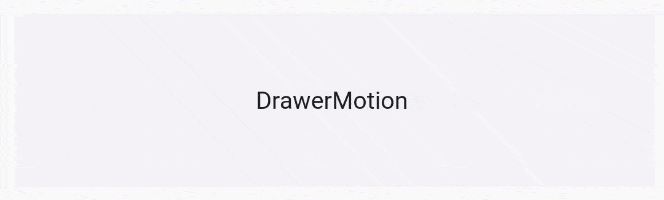
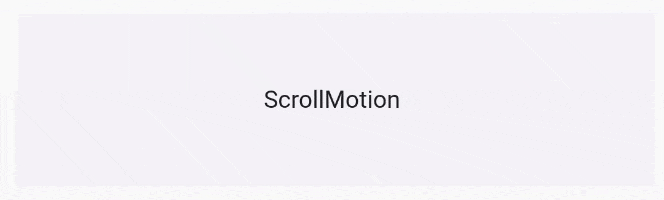
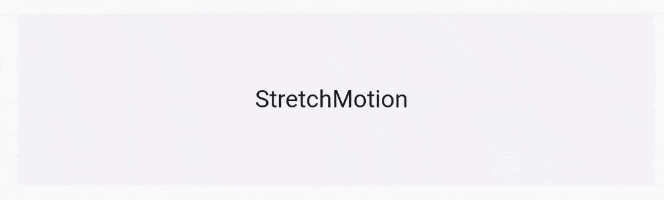
Conclusion
WRITTEN BY
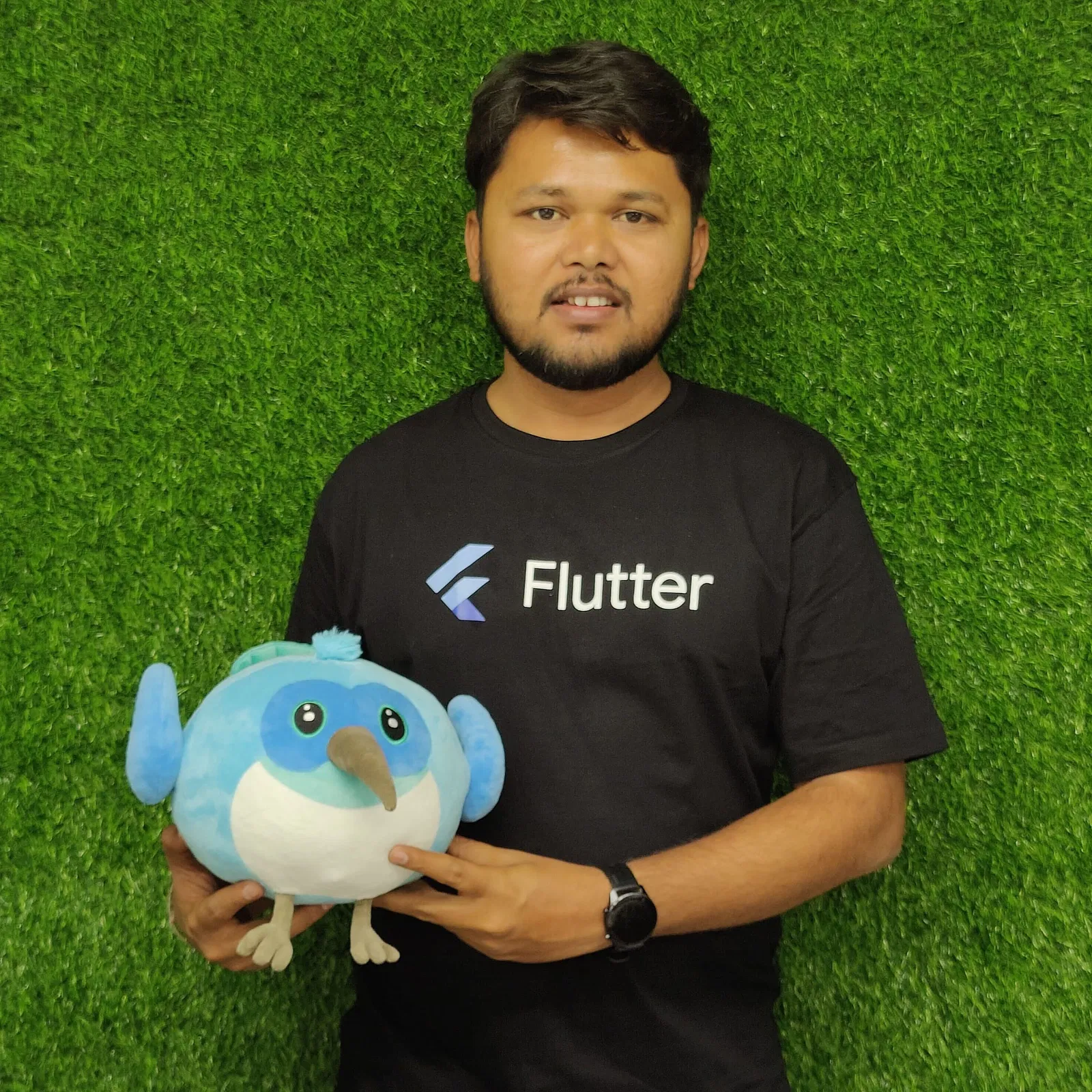
Pratik Butani
Pratik is a mobile app developer who makes both iOS and Android apps look and feel amazing using Flutter. He brings ideas to life with clean, smooth, and functional apps that users love.
WRITTEN BY
Pratik Butani
Pratik is a mobile app developer who makes both iOS and Android apps look and feel amazing using Flutter. He brings ideas to life with clean, smooth, and functional apps that users love.
More
An interesting read? Here is more related to it.
10 September 2024
Hemratna Bhimani24 September 2024
Mukund JogiMaking IT Possible
Making IT Possible
Making IT Possible
Making IT Possible
Making IT Possible
Making IT Possible
India (HQ)
201, iSquare Corporate Park, Science City Road, Ahmedabad-380060, Gujarat, India
For Sales
[email protected]
Looking For Jobs
Apply Now